Java SE 8: Getting Started with String Processing
Overview
Purpose
This tutorial shows you how to use string processing in Java Platform, Standard Edition 8 (Java SE 8) and NetBeans 8.
Time to Complete
Approximately 80 minutes
Introduction
In this tutorial, you learn how to deploy and run a Java application so that you can use the String, StringBuffer, StringBuilder, StringTokenizer, and Scanner Java classes. You also learn about each class's representation, methods, and advantages. You use:- String class methods to manipulate common string operations. You learn to convert all characters in a string to uppercase and lowercase, trim the string to remove whitespace, and retrieve a substring from a string.
- StringBuilder class methods like insert(), delete(), reverse(), length(), and append() to improve the application performance of single-threaded applications.
- StringTokenizer class to create an application where you break a string into tokens. You apply different constructors to create a StringTokenizer instance and specify different types of delimiters to retrieve tokens.
- Scanner class to create an application that accepts user input from the console and breaks the string into tokens. Using a delimiter pattern, which by default matches the whitespace, you learn how to convert the resulting tokens into values of different data types, and you retrieve the tokens with various methods.
Hardware and Software Requirements
Creating a Java Application
In this section, you create a Java application to demonstrate string processing in Java.
-
Select File > New Project to open NetBeans IDE 8.0.
- Perform the following steps on the Choose Project page:
- Select Java from Categories.
- Select Java Application from Projects.
- Click Next.
- Perform the following steps on the Name and Location page:
- Enter StringsDemo as the project name.
- Select Create Main Class.
- Enter the following:
- Package name: com.example.strings
- Class name: StringsMethods
- Click Finish.
You successfully created a Java application with StringsMethods.java.
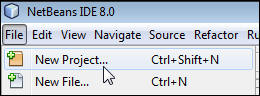
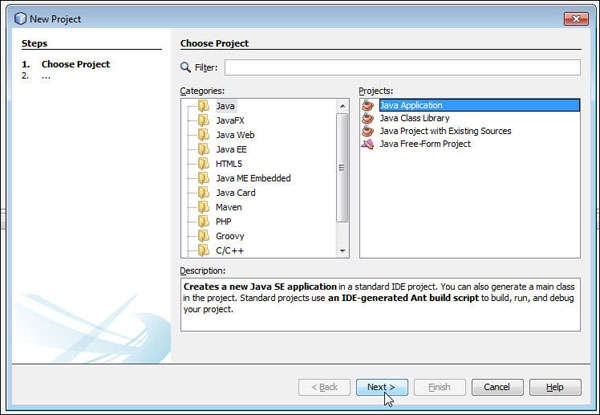
Invoking the String Class Methods
String is one of the most widely used classes in Java due to its unique characteristics (for example, the Java String is immutable and final by default). Using the String class in multithreaded environment is safe because the class takes care of the precautionary measures.
Consider the following scenarios, where you need to manipulate the strings in the applications:
- Enter data while filling the application form. You enter name, date of birth, address, phone number, email address, and other details.
- Use strings to match other strings; for example, to find all people whose last name begins with "Smith."
- Break a string and create multiple strings. For example, "Michael Thomas Smith, 101 Main Street, Boston, MA, 01234 " is a concatenation of First Name, Last Name, Street, City, State, and Zip.
- Parse a string with delimiters into tokens.
- Mix different data types. For example, "Michael Thomas Smith, 28, 06-apr-1986, 1234, 3500000" is a combination of String, int, Date, int, and float data types.
- To evaluate a string for a match against another string, alter the initial string. For example, to match any name in the database against "smith," eliminate the whitespace and change the case to uppercase or lowercase.
- Use only part of a string to accommodate character limits. For example, if the field length is 10 characters, reduce the "Michael Thomas Smith" string to accept the first 10 characters.
Note: String class objects are immutable; that is, when you change a string, a new object is created, the old object is no longer used, and there is increased garbage collection of string objects. StringBuffer and StringBuilder class objects are mutable; that is, when you change a string object, you can manipulate the string without creating a new object.
In this section, you modify the StringMethods class to invoke the String class methods.
-
In the NetBeans IDE, perform the following steps:
- Expand
Source Packages > com.example.strings.
- Open the provided StringsDemo project.
- On the Projects tab, double-click StringsMethods.java.
- Expand
-
Open StringsMethods.java and perform the following steps:
-
Initialize the following string variable: String sentence = "The String Class represents character Strings. ";
-
Add the toUpperCase()method and use the toUpperCase method to change the same string to uppercase. Locate the appropriate comment to add the code.
-
For the sentence variable, use the trim()method to remove the extra spaces.
-
Use the length()method to retrieve the length of the string.
-
contains(): Returns true if and only if this string contains the specified sequence of char values; otherwise, returns false.
- toLowerCase(): Converts all string characters to lowercase.
- toUpperCase(): Converts all string characters to uppercase.
- equals(): Compares string to the specified object.
- equalsIgnoreCase(): Compares string to another string, ignoring case considerations.
- substring(): Returns a new string that is a substring of the given string.
- compareTo(): Compares the character sequence represented by the string object to the character sequence represented by the argument string.
- trim(): Returns a copy of the string with leading and trailing whitespace omitted.
- length(): Returns the length of the string.
- replace(): Returns a new string resulting from replacing all occurrences of oldChar in the string with newChar.
-
On the Projects tab, right-click StringsMethods.java and select Run File.
- Verify the output.
package com.example.strings;
public class StringsMethods {
public static void main(String[] args) {
//Initialize the String variable as
mentioned
boolean sentenceContainsClass =
sentence.contains("Class");
System.out.println("Does the sentence
contain 'Class': " + sentenceContainsClass);
sentence = sentence.toLowerCase();
System.out.println("Lower case stmt :" +
sentence);
//Your code goes here. Convert the string
to uppercase and print on the console
String s1 = "class", s2 = "Class";
boolean s = s1.equals(s2);
System.out.println(".equals returns: " +
s);
boolean strIgnore =
s1.equalsIgnoreCase(s2);
System.out.println(".equalsIgnoreCase
returns: " + strIgnore);
String aNewString = sentence.substring(8,
49);
System.out.println("Substring is :"
+ aNewString);
String str1 = "Oracle",str2 =
"Corporation";
int retval = str1.compareTo(str2);
if (retval < 0) {
System.out.println("str1 is greater than str2");
} else if (retval == 0) {
System.out.println("str1 is equal to str2");
} else {
System.out.println("str1 is less than str2");
}
//Your code goes here. Trim the string
variable by removing extra space and print on the console
//Your code goes here. Get the length of
the string and print on the console
String r = aNewString.replace('E', 'Y');
System.out.println("Replace of char'E'
with 'Y': " + r);
}
}

Here are descriptions of the methods:
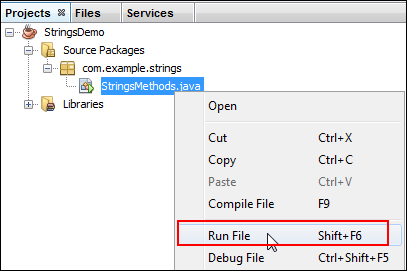
Working with the String, StringBuffer, and StringBuilder Classes
In this section, you compare the String, StringBuffer, and StringBuilder classes. You edit Stringcomparison.java to understand the usage and working style of different string classes using the concat() method. You also learn about the StringBuilder class methods.
Using String-Related Classes
-
In the NetBeans IDE, perform the following steps:
- Expand
Source Packages > com.example.strings
. - Double-click the provided StringsDemo project.
- On the Projects tab, create a Java file named Stringcomparison.java.
- Expand
-
Open Stringcomparison.java in the code editor window, and then perform the following steps:
- Assign and declare the Stringcomparison
class variables.
- Create an instance of the Stringcomparison class and invoke the String, StringBuffer, and StringBuilder class concatenation methods.
- Generate the concat(),
concat1(), and concat2()methods to perform string
concatenation for the String,
StringBuffer,
and
StringBuilder classes, respectively.
package com.example.strings;
public class Stringcomparison {
public static void main(String[] args) {
String s1 = "Java";
String s2 = "Oracle";
Stringcomparison sc = new Stringcomparison();
sc.concat(s1, s2);
sc.concat1(s1, s2);
sc.concat2(s1, s2);
}
public String concat(String s1, String s2) {
System.out.println("String concatenation " + (s1 + s2));
return s1 + s2;
}
public String concat1(String s1, String s2) {
StringBuffer sb = new StringBuffer();
sb.append(s1);
sb.append(s2);
System.out.println("String Buffer concatenation " + sb);
return sb.toString();
}
public String concat2(String s1, String s2) {
StringBuilder sb1 = new StringBuilder();
sb1.append(s1);
sb1.append(s2);
System.out.println("String Builder concatenation " + sb1);
return sb1.toString();
}
} -
On the Projects tab, right-click
Stringcomparison.java
and select Run File. - Verify the output.
The code demonstrates how to use the String, StringBuffer, and StringBuilder class methods to perform string concatenation. -
Edit the main()method in Stringcomparison.java to understand the usage of the insert()and append()methods in the string concatenation process.
// preexisting code goes here
System.out.println("StringBuilder insert and append example!");
StringBuilder sb = new StringBuilder(0);
System.out.println(sb.insert(0, "101 street"));
int len = sb.length();
System.out.println(sb.insert(len, "Boston"));
System.out.println(sb.insert(10, "/"));
System.out.println(sb.append("United states"));The code creates an instance of the StringBuilder class, which provides the insert() and append()methods.
-
insert(): Inserts the second argument in the string builder. This method lets you add data in any position.
Syntax: insert(int pos, String str) where
pos is the position where the data will be inserted.
str is the string that will be inserted. The data is converted to a string before the insert operation takes place. - length(): Returns the length of the string.
-
append(): Appends the argument to this string builder. The method appends the data at the last position.
Syntax: append(String str) where
str is the string to be appended.
Note: Use concatenation to attach a string at the end of another string. However, use the append() method with the StringBuffer or StringBuilder class to append character sequence, boolean, float, int, object, or string.
-
-
On the Projects tab, right-click Stringcomparison.java and select Run File.
- Verify the output.
You successfully used the insert() and append()methods in the StringBuider class.
Here are the descriptions of the classes:
String class: When you performed string concatenation in JDK version 1.1, the String class created three string objects in the heap. Of the three string objects, two object references were lost and was garbage collected. If the number of strings for concatenation increased by 10, 20, and so on, intermediate string references were created, and the heap memory was occupied for garbage collection. To solve the problem, JDK version 1.3 introduced the StringBuffer class.
StringBuffer class: You use the StringBuffer class for multithreaded applications. Every string buffer has a capacity. As long as the length of the character sequence in the string buffer doesn't exceed the capacity of 2^31-1, you don't have to allocate a new internal buffer array. If you increase the maximum number of elements in the array, the array goes to negative and throws an error. String buffers are safe to use when multiple threads are involved in the application. In the JDK 5 release, the StringBuffer class was supplemented with an equivalent class, StringBuilder, which was designed for single-thread applications.
StringBuilder class:
In general you should use the StringBuilder
class instead of the StringBuffer class.
The StringBuilder class
executes faster, uses memory more efficiently, and
provides better performance when you must make many
concatenations. The StringBuilder class
has no array size limit and supports all StringBuffer class
methods. When you perform string concatenation with the +
operator, Java internally converts that call to the
corresponding StringBuilder
append() method.
For example, implementation of return
s1+s2; in the String
class is equivalent to return
new StringBuilder().append(s1).append(s2).toString();
in the StringBuilder
class.


Using StringBuilder Class Methods
- In the NetBeans IDE, perform the following steps:
- Expand
Source Packages > com.example.strings.
- Open the provided StringsDemo project.
- On the Projects tab, create a Java file named StringBuildermethod.java.
- Expand
- Open
StringBuildermethod.java in the code editor window.
Add the following code to
the main()
method: -
On the Projects tab, right-click StringBuildermethod.java and select Run File.
-
Verify the output.
In this section, you use the StringBuilder
class methods. The StringBuilder
class objects let you manipulate strings without creating a
new object. They are unsynchronized (that is, the
StringBuilder
class isn't used for multithreaded
applications). The StringBuilder
class is designed for use as a drop-in replacement for the StringBuffer class.
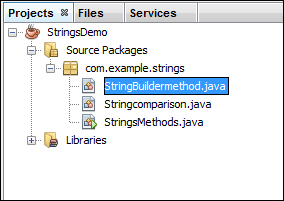
public static void main(String[]
args) {
StringBuilder sb1 = new
StringBuilder("Welcome to the Java World");
sb1.delete(11, 14);
System.out.println("Delete method
demo: " + sb1);
StringBuilder sb2 = new
StringBuilder(sb1);
sb2.insert(11, "Sun ");
System.out.println("Insert Operation:
" + sb2);
StringBuilder sb3 = new
StringBuilder(sb2);
sb3.replace(11, 14, "oracle");
System.out.println("Replace
Operation: " + sb3);
StringBuilder sb4 = new
StringBuilder(sb3);
sb4.setCharAt(11, 'O');
System.out.println("Replacing char at
index 11 with Caps O: " + sb4);
StringBuilder sb5 = new
StringBuilder(sb4);
sb5.reverse();
System.out.println("Reverse of
Welcome to oracle Java World : " + sb5);
int length= sb5.length();
System.out.println("Length of the
string:" +length);
}
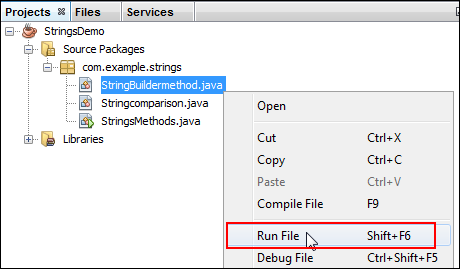
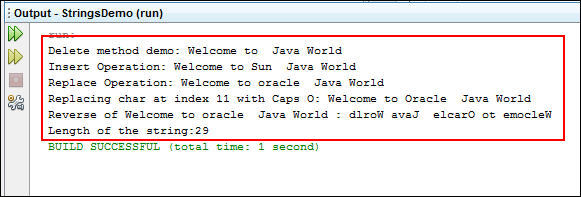
You successfully used the StringBuider class methods.
Working with the String Tokenizer Class
split()
and
append()
methods with the StringBuilder
class. Retrieving Tokens Using the StringTokenizer Class
You use the StringTokenizer class to read tokens directly from a file. Reading tokens makes some utilities efficient because they work with individual tokens rather than lines of text. The StringTokenizer class methods don't distinguish among identifiers, numbers, and quoted strings, and they don't recognize and skip comments.
You create the class object by using one of the following constructor methods:
- If a string is passed as a single parameter to the constructor, the source text is broken by a default set of delimiters, such as space, tab, newline, cr, or formfeed (whitespace). Syntax: StringTokenizer(String str)
- If a second string is also passed, it is assumed to be a set of delimiting characters. Syntax: StringTokenizer(String str,String delim)
- If you add a true
flag as a third parameter, delimiters are also
returned as string tokens. Syntax: StringTokenizer(String
str,String delim,boolean
returnDelims)
In this example, you apply the tokenizer function on a URL. You also learn how to break the URL based on different delimiters or use the StringTokenizer class to break characters.
-
In the NetBeans IDE, perform the following steps:
- Expand
Source Packages > com.example.strings.
- Open the provided StringsDemo project.
- On the Projects tab, create a Java file named Tokenizer.java.
- Expand
-
Open Tokenizer.java in the code editor window. Add the following code to the main() method, and then press Ctrl + Shift + I to solve the imports.
String url = "http://192.173.15.36:8084/";
StringTokenizer st = new StringTokenizer(url);
int tokenCount = st.countTokens();
while (st.hasMoreTokens())
{
System.out.println(st.nextToken());
}
System.out.println("Number of tokens generated using single parameter constructor = " + tokenCount);
-
On the Projects tab, right-click Tokenizer.java and select Run File.
- Verify the output.
-
In the main()method, add the following code to demonstrate the two-argument constructor:
StringTokenizer str = new StringTokenizer(url, ":/.");
int tokenCounts = str.countTokens();
while (str.hasMoreTokens()) {
System.out.println(str.nextToken());
}
System.out.println("Number of tokens generated using two parameter constructor = " + tokenCounts);
-
On the Projects tab, right-click Tokenizer.java and select Run File.
- Verify the output.
-
In the main()method, add the following code to demonstrate the three-argument constructor:
StringTokenizer str1 = new StringTokenizer(url, ":/.", true);
int tc = str1.countTokens();
while (str1.hasMoreTokens()) {
System.out.println(str1.nextToken());
}
System.out.println("Number of tokens generated using three parameter constructor = " + tc);
-
On the Projects tab, right-click Tokenizer.java and select Run File.
-
Verify the output.
This code sets the URL to the string variable message, gets the instance of the StringTokenizer object, retrieves the number of tokens, and displays the number of generated tokens in the console.
For the single parameter constructor, this example demonstrates where you break the source text at the default set of delimiters (for example, whitespace and newline).
This code generates the number of tokens based on the delimiting character passed in the second argument. The number of generated tokens is displayed in the console.
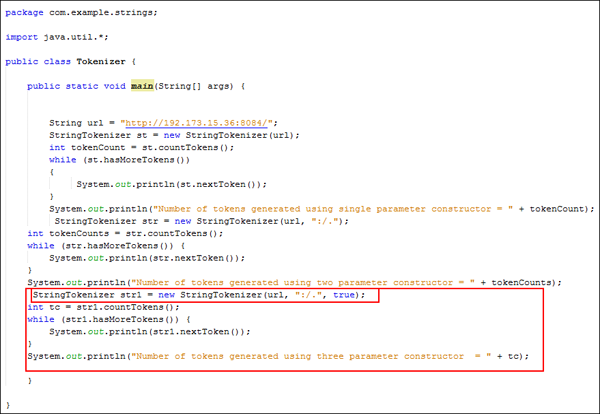
This code generates the number of tokens based on the
delimiting character passed in the second argument. When you
set the third parameter to true
, the parameter
returns the string token and any delimiters.
Retrieving the Address List Using the split() and append() Methods
In this section, you use the split() method in Address.java to retrieve the address from the address array list and display the address in the console.
-
In the NetBeans IDE, perform the following steps:
- Expand
Source Packages > com.example.strings.
- Open the provided StringsDemo project.
- On the Projects tab, create a Java file named Address.java.
- Expand
-
Open Address.java in the code editor window and then perform the following steps:
- Declare the Address
class variables.
- Generate the getter methods for each variable.
- Generate the parametrized constructor.
- Override the
toString()
Address.java
to display theAddress
object in the console.// preexisting code goes here
@Override
public String toString() {
StringBuilder sb = new StringBuilder(256);
sb.append("Door ID: ").append(this.getDoorNo()).append("\n");
sb.append("Street: ").append(this.getStreet()).append("\n");
sb.append("City: ").append(this.getCity()).append("\n");
sb.append("Country: ").append(this.getCountry()).append("\n");
return sb.toString();
}StringBuilder
class. AStringBuilder
class provides theappend() method, which appends the field values
to the fields. You use thethis()
object reference to retrieve the values. -
Use
the split()
method to retrieve the addressList address array list. - Add the following code to the
main()
method:
public static void main(String[] args) {
String[] add = new String[6];
List<Address> addressList = new ArrayList<>(11);
add[0] = "No20,MG Road,Bangalore,India";
add[1] = "No657,Maple Street,Redwood City(CA),USA";
add[2] = "No67,Oak Street,Colombo,Srilanka";
add[3] = "No54,Lake Street,Bangkok,Thailand";
add[4] = "No122,Washington Street,Tokyo,Japan";
add[5] = "No673,Hill Street,Melbourne,Australia";
for (String curLine : add) {
String[] e = curLine.split(",");
addressList.add(new Address(e[0], e[1], e[2], e[3]));
}
System.out.println("=== Address List ===");
for (Address address : addressList) {
System.out.println(address.toString());
}
} - Press Ctrl + Shift + I to solve the imports.
-
On the Projects tab, right-click Address.java and select Run File.
-
Verify the output.
package
com.example.strings;
public class Address {
private String doorNo = "";
private String street = "";
private String city = "";
private String country = "";
public String getDoorNo() {
return this.doorNo;
}
public String getStreet() {
return street;
}
public String getCity() {
return city;
}
public String getCountry() {
return country;
}
public Address(String doorNo, String
street, String city, String country) {
this.doorNo = doorNo;
this.street = street;
this.city = city;
this.country = country;
}
}
The for each loop retrieves the individual addressList array objects, and the toString() method displays the objects in the console.

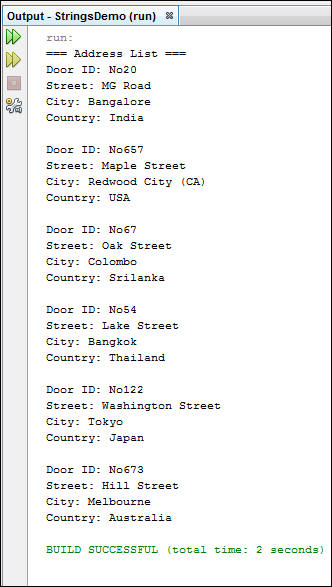
The String.split() syntax is easier than the StringTokenizer syntax. However, if you want to parse the resulting strings, or if you want to change the delimiter based on a particular token, String.split() won't help because it returns an array (String[]), whereas StringTokenizer returns one token at a time.
The StringTokenizer class removes tokens that are delimited by fixed substrings. As a result, StringTokenizer is about twice as fast as String.split(). You can also use different tokens with the same StringTokenizer object. You cannot do this with the split() method because, after the array is returned, it can’t be reused for another delimiter.
Working with the Scanner Class
The Scanner class was introduced JDK 1.5 and is the equivalent of the C language scanf function. This class breaks the input into tokens by using a delimiter pattern, which by default matches the whitespace. The resulting tokens can then be converted into values of different types. A simple text scanner can use regular expressions to parse primitive types and strings.
Note: The Scanner class isn't safe for multithreaded environment. Use it without external synchronization.
Consider a school management system, where instructors must enter student details like rollno, name, marks for each subject, and the total marks. In this section, you use the Scanner class to accept user input from the command line or the console.
-
In the NetBeans IDE, perform the following steps:
- Expand
Source Packages > com.example.strings.
- Open the provided StringsDemo project.
- On the Projects tab, create a Java file named ScannerDemo.java.
- Expand
-
Open ScannerDemo.java in the code editor window. Add the following declaration inside the ScannerDemo class:
private static int mathmarks;
private static int sciencemarks;
This code initializes the mathmarks and sciencemarks integer variables.
-
In the main()method, add the following code to enter student curriculum details and calculate the total marks obtained:
-
Press Ctrl + Shift + I to import the Scanner class.
-
nextDouble(): Scans the input's next token as a double.
-
next(): Finds and returns the next complete token from this scanner.
- nextInt():
Scans the input's next token as an
int.
-
nextBoolean(): Scans the input's next token into a boolean value and returns that value.
-
On the Projects tab, right-click ScannerDemo.java and select Run File.
-
Verify the output.
package com.example.strings;
import java.util.Scanner;
public class ScannerDemo {
private static int mathmarks;
private static int sciencemarks;
public static void main(String args[]) {
Scanner scanner = new Scanner(System.in);
System.out.println("Enter your rollno");
double rollno =
scanner.nextDouble();
System.out.println("Enter your name");
String name = scanner.next();
boolean option = false;
while (true) {
System.out.println("\nEnter Marks Obtained\n");
System.out.println("(1) - Maths");
System.out.println("(2) - Science");
System.out.println("(3) - Quit");
System.out.print("Please enter your selection:\t");
int selection =
scanner.nextInt();
if (selection == 1) {
System.out.print("Enter Marks of Maths\t");
mathmarks =
scanner.nextInt();
} else if (selection
== 2) {
System.out.print("Enter Marks of Science\t");
sciencemarks =
scanner.nextInt();
} else if (selection
== 3) {
System.out.print("Sure
u want to Quit : True or False \t");
try {
option = scanner.nextBoolean();
if
(option) {
System.out.println("Thank you for using the student
portal");
break;
}
else {
System.out.println("Log in again to use the student
portal");
break;
}
} catch
(java.util.InputMismatchException exception) {
System.out.println("Invalid input. Exiting");
break;
}
}
}
int total = mathmarks + sciencemarks;
System.out.println("********** Student
details **********");
System.out.println("Rollno:" + rollno +
"\nName:" + name + " \nMaths Marks:" + mathmarks +
"\nScience Marks:" + sciencemarks);
System.out.println("Total marks: " +
total);
}
}
This code returns the value of the student roll number, name, marks for maths and science, and total marks of both. Using the scanner class methods, you retrieve the values and display them in the console.

Here are the descriptions of the methods:

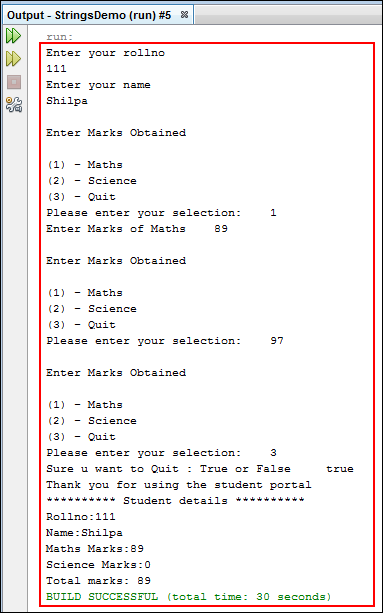
Summary
In this tutorial, you learned how to use the following string-related classes:
- String
- StringBuilder
- StringTokenizer
- Scanner
Resources
For more information about the topics, refer:
Java SE 8 documentation on String, StringBuilder, StringTokenizer , and Scanner.To learn more about Java SE, refer to additional OBEs in the Oracle Learning Library.
Credits
- Curriculum Developer: Shilpa Chetan
To navigate this Oracle by Example tutorial, note the following:
- Topic List:
- Click a topic to navigate to that section.
- Expand All Topics:
- Click the button to show or hide the details for the sections. By default, all topics are collapsed.
- Hide All Images:
- Click the button to show or hide the screenshots. By default, all images are displayed.
- Print:
- Click the button to print the content. The content that is currently displayed or hidden is printed.
To navigate to a particular section in this tutorial, select the topic from the list.